Algorithm 1: Grey scale, negative
This very simple test demonstrates grey scale and negative of input images. Grey scaling calculate length of RGB vector then constructs vector with the same length but R, G, B components have the same length each. Negative uses fact that max R, G, or B value is 255 and calculates difference between color component and 255.see:
test01.scm
(define b (img_open "in/ngirl.jpg")) (img_gr b) (img_neg b) (img_save b "out/gr.jpg")
Input images:
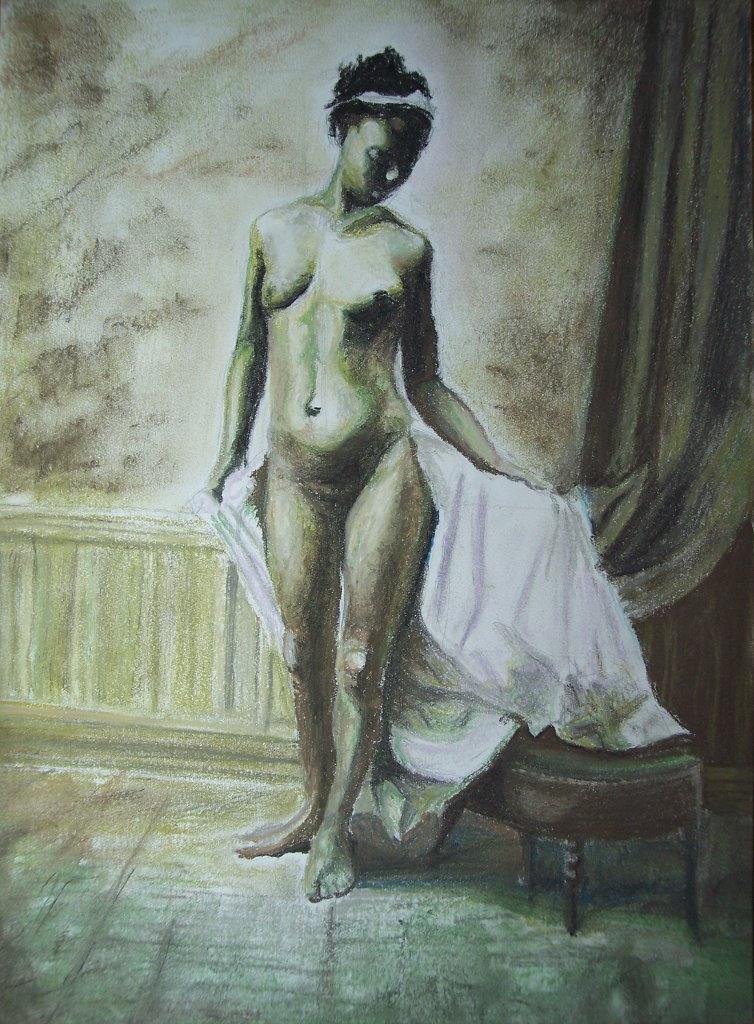
Output images:
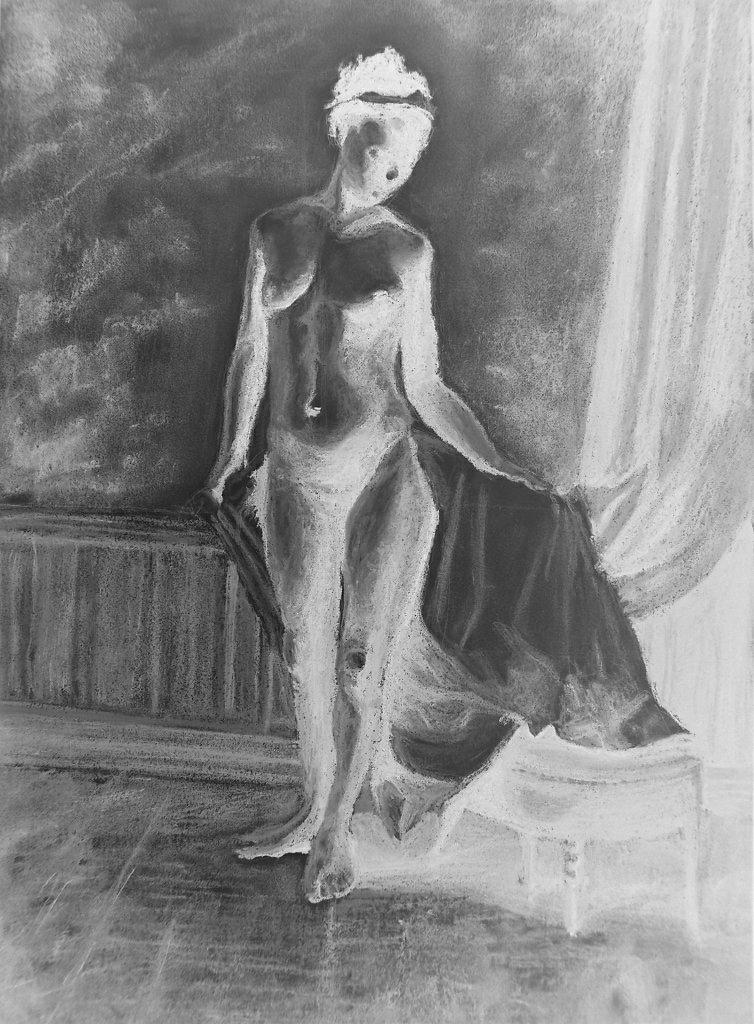
Algorithm 2: Emboss
It's classical image processing method: applying of 2D filter on each pixel. More about convolution matrix read: this. Main idea is the same as in other filters: each original samples gives some value to common result but all of thees values has different amount which depend of filter formula. There are 2 main notes on this filter. First: no recursion, so original pixels (samples) - are real original and temporary clean copy of original image should be allocated and used for calculation. Second: normalization of result is need. Result is divided by special value (div) and shifted by another (shift or bias). And if now it's lesser then 0, 0 should be used instead of, if bigger then 255, 255 should be placed as result. This test uses convolution matrix to implement emboss filter. Other tests use c.m. for other goals.see:
test02.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_conv b emboss-matrix emboss-div emboss-shift) (img_save b "out/emboss.jpg")
Input images:
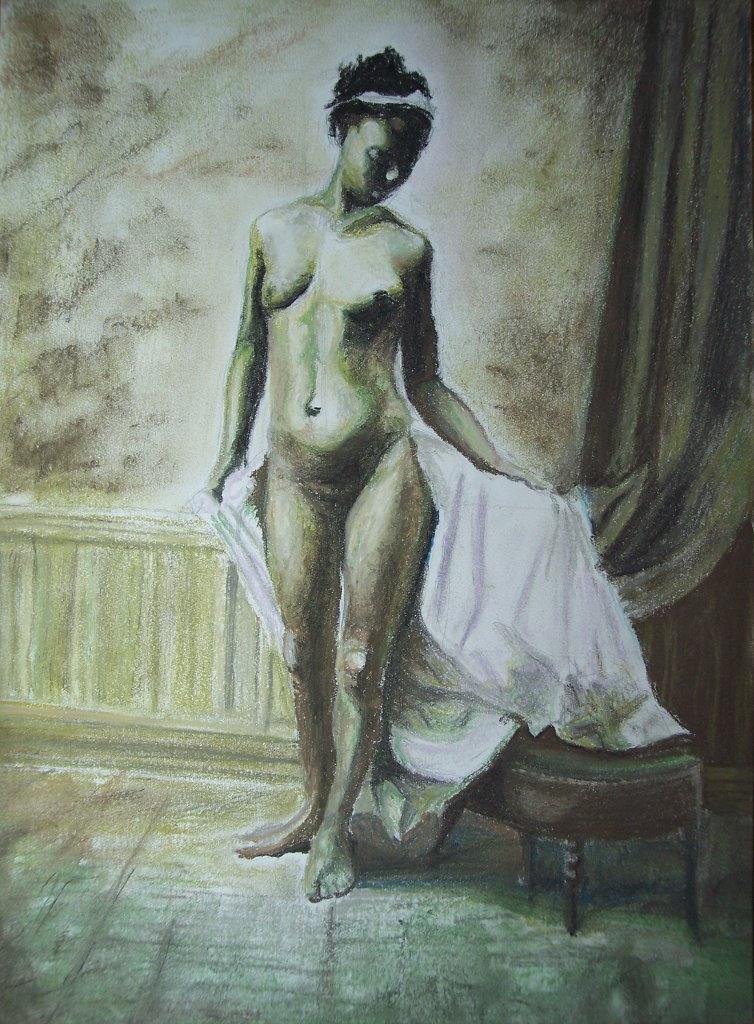
Output images:
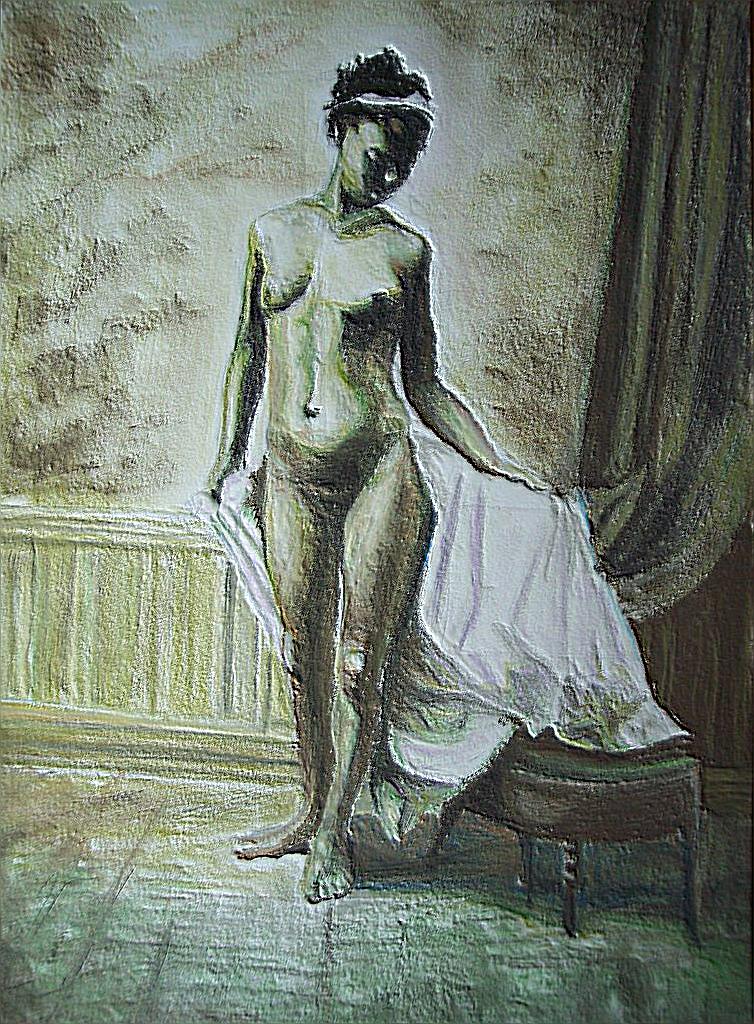
Algorithm 3: Color mask
This is also simple algorithm which applies color mask on image: with 3 bits user select one of 3 channels (R, G, B) to show, other will be hidden. This looks like broken TV when picture is blown in some color (green often). For example, bits "3" means "011", i.e. R channel will be hidden, G, B - shown.see:
test03.scm
(define b (img_open "in/ngirl.jpg")) (img_ch b 3) (img_save b "out/ch.jpg")
Input images:
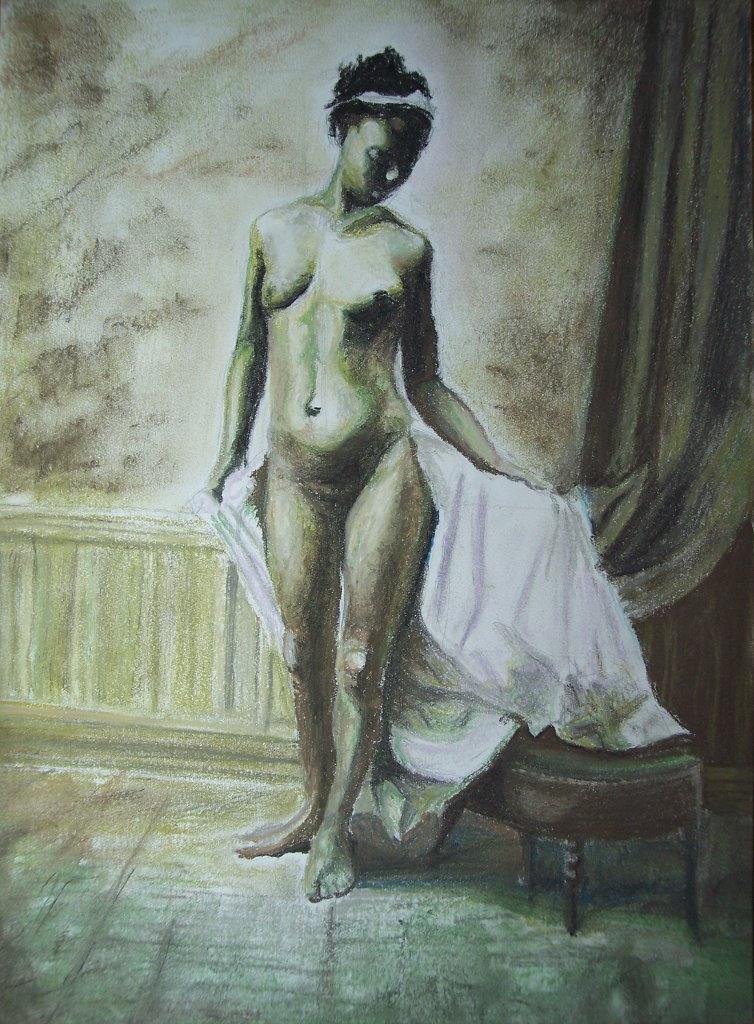
Output images:
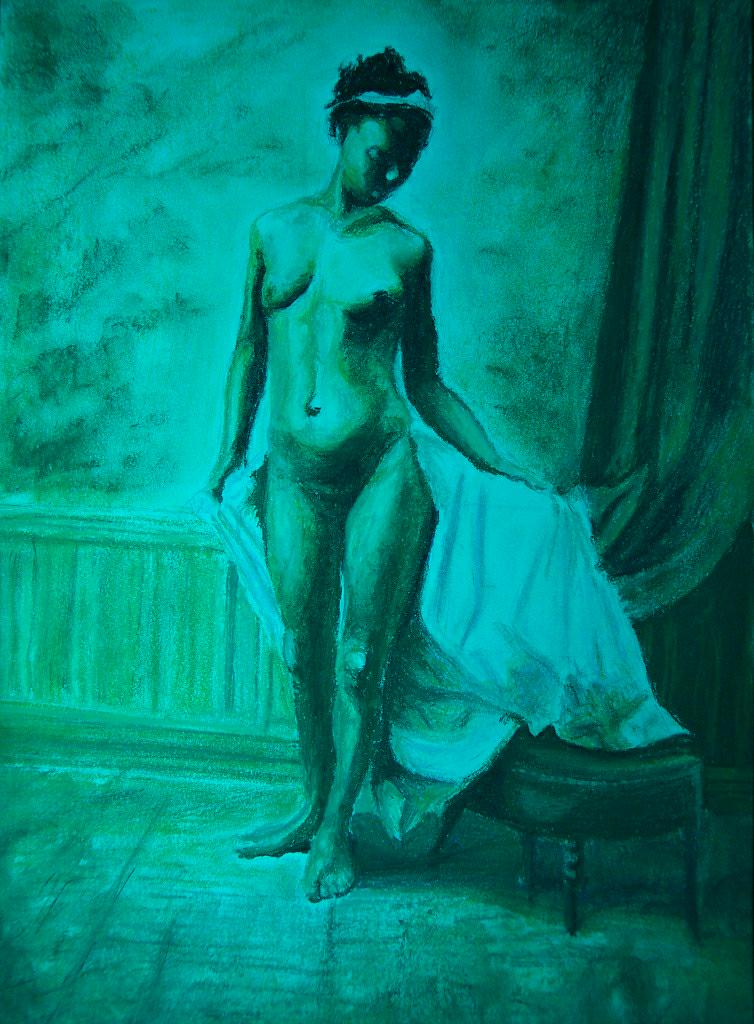
Algorithm 4: Edge detection
This test uses convolution matrix to implement edge detection of image. There are different algorithms for edge detection also, not only based on c.m.see:
test04.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_conv b edge-matrix 3 60) (img_save b "out/edge.jpg")
Input images:
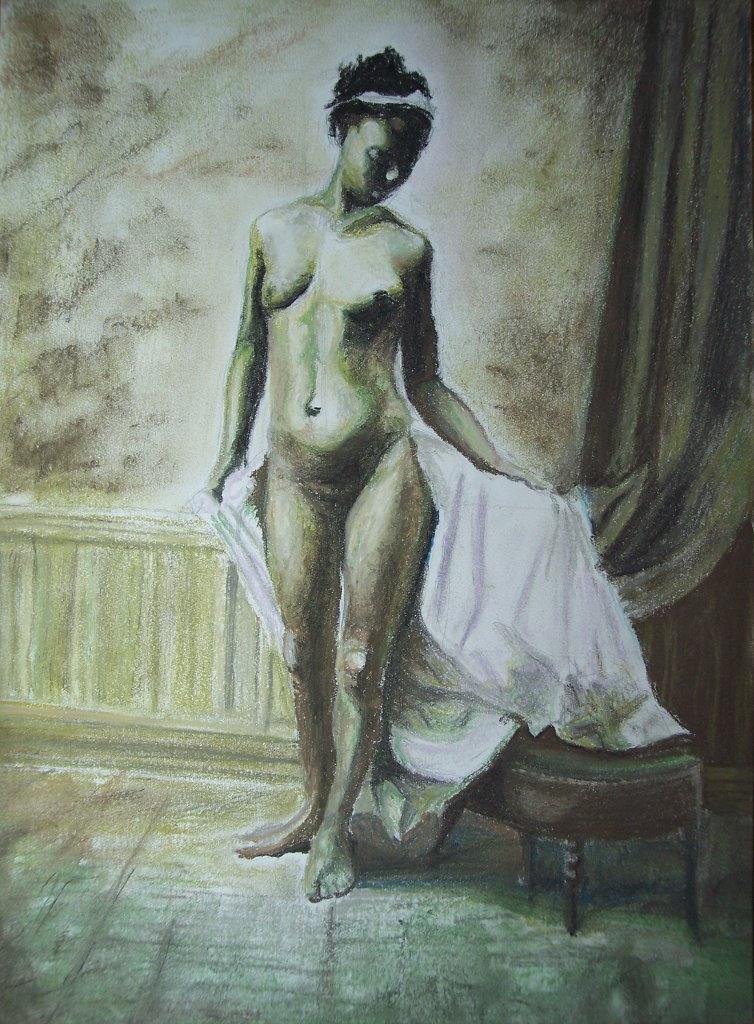
Output images:
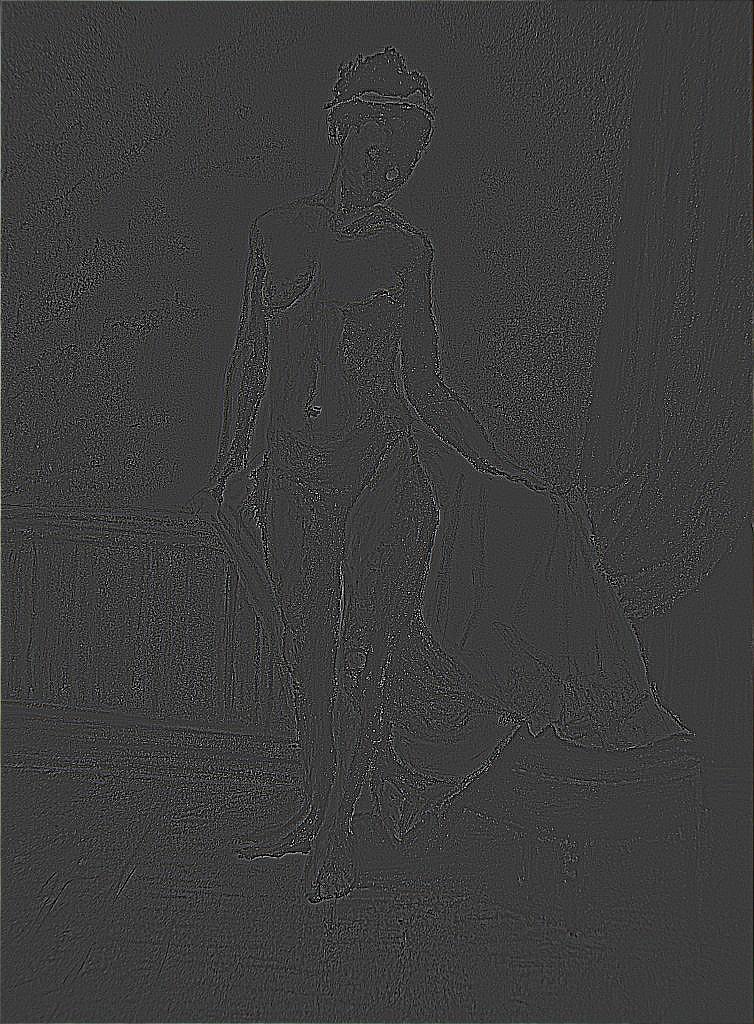
Algorithm 5: Grey levels
This test implements converting to grey levels with custom number of levels. Resulting image looks like picture from Windows 3.1 - colors are approximated with grey levels only. When there are only 2 levels, all looks like black-and-white image, more levels show more realistic graduations of colors.see:
test05.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_grl b 6) (img_save b "out/grl.jpg")
Input images:
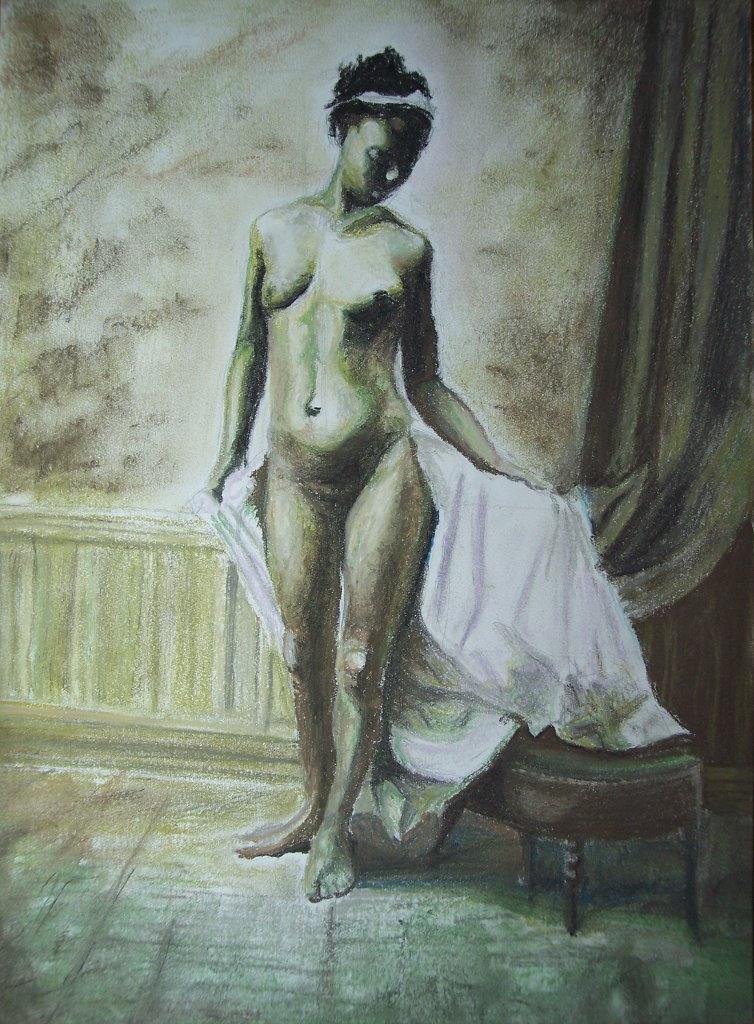
Output images:
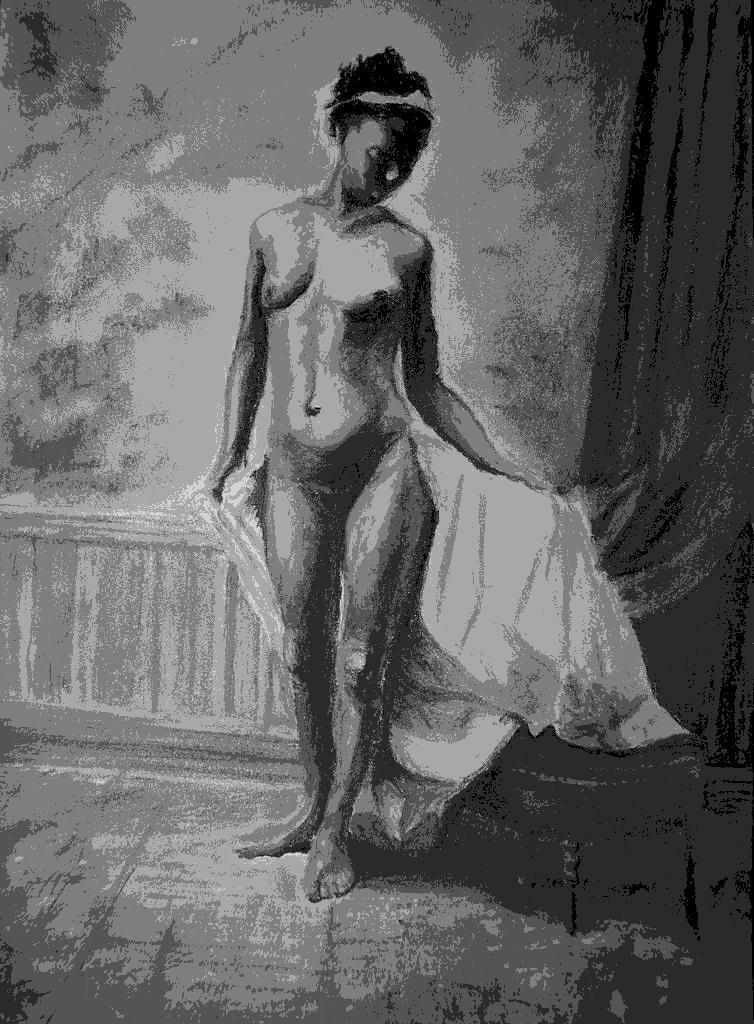
Algorithm 6: B/w conversion + edge detection
This test runs edge detection with c.m. too, but image is converted to black-and-white first.see:
test06.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_bw b 50) (img_conv b edge-matrix 3 40) (img_save b "out/bwedge.jpg")
Input images:
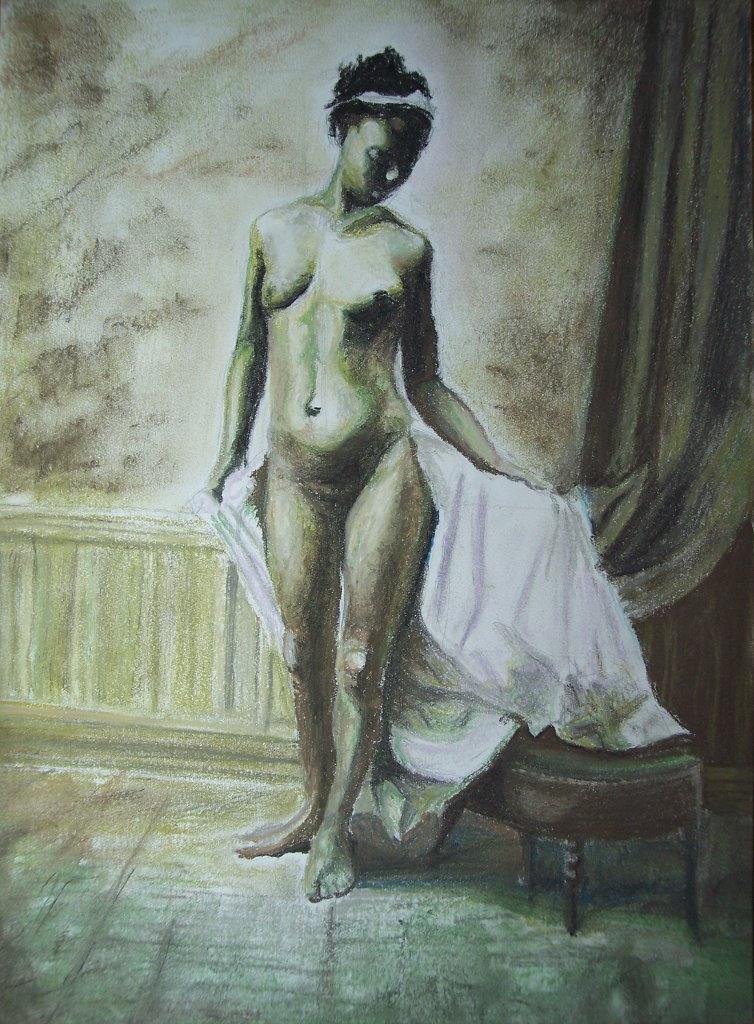
Output images:
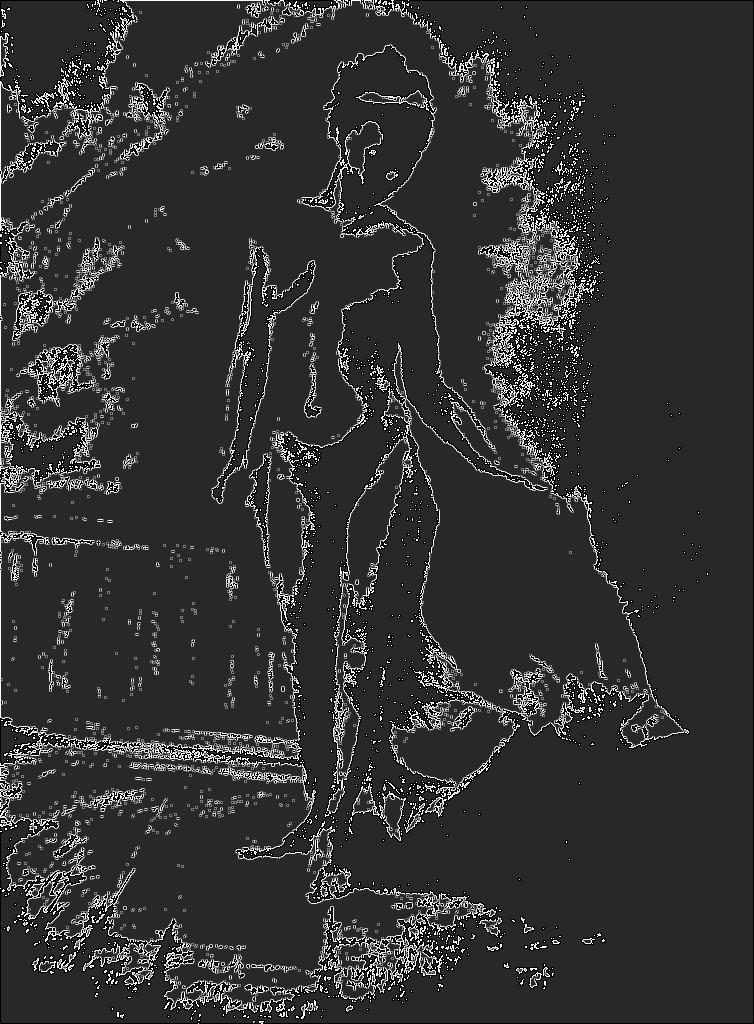
Algorithm 7: B/w conversion + dilation
This test converts input image to black-and-white then applies dilation matrix (which is c.m. too).see:
test07.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_bw b 60) (img_conv b dilat-matrix dilat-div dilat-shift) (img_conv b dilat-matrix dilat-div dilat-shift) (img_save b "out/dilat2.jpg")
Input images:
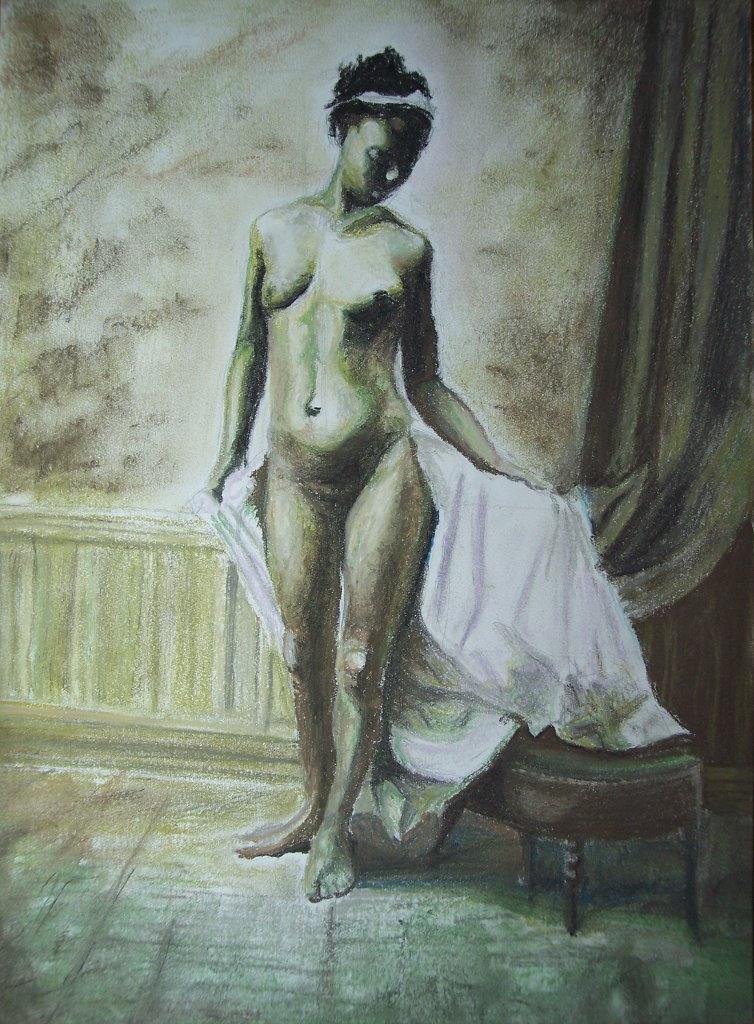
Output images:
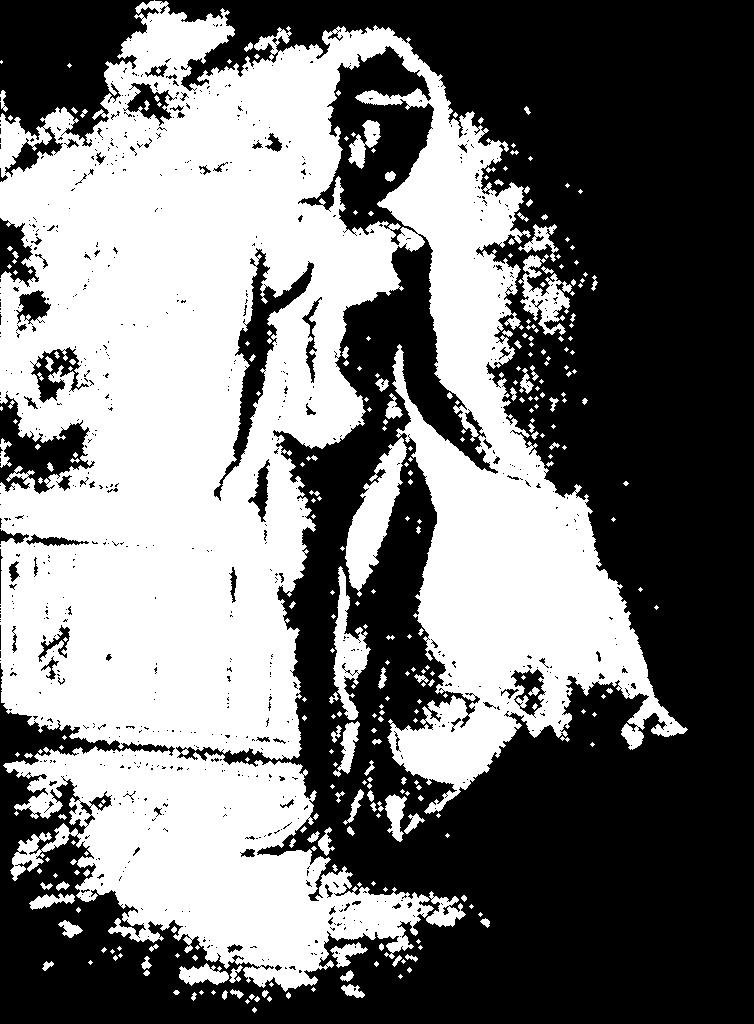
Algorithm 8: Pixalization
This test runs pixalization. Main idea is to cut original image on squares with some side and to replace pixels of this squared area with one-color square. Which color to use? If black and white version is applied then result color is calculated like in Black-and-White filter with threshold = 50%. If color version of pixalization is applied, then average color of squared area is used.see:
test08.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_pix b 10 1) (img_save b "out/pix1.jpg") (define b (img_open "in/ngirl.jpg")) (img_pix b 5 0) (img_save b "out/pix2.jpg")
Input images:
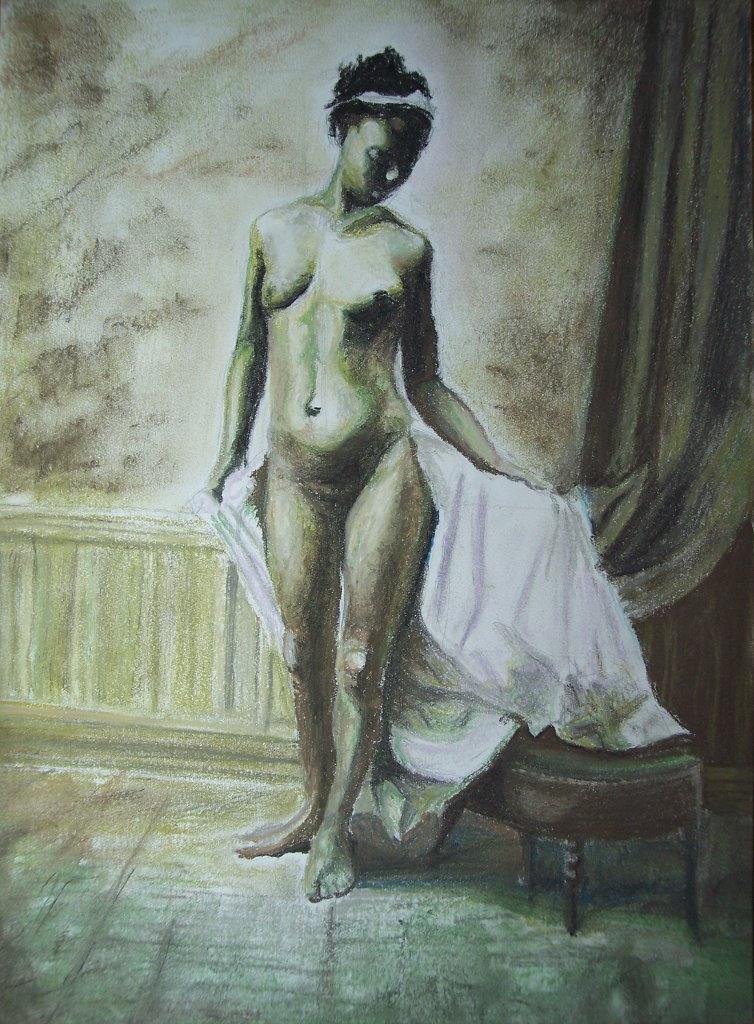
Output images:
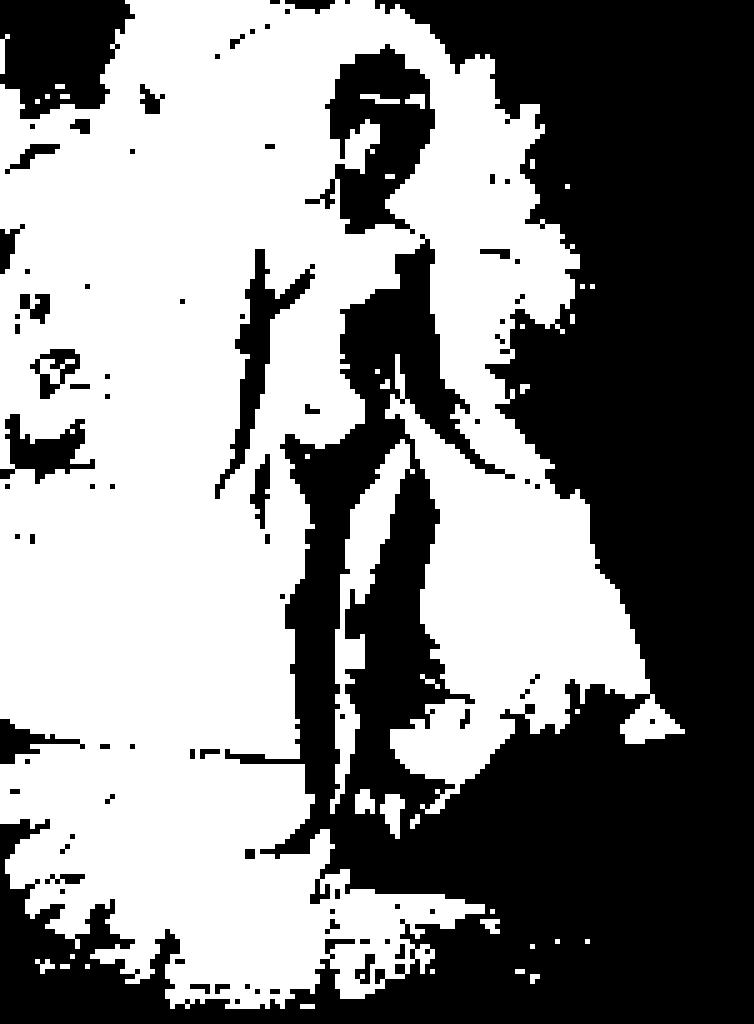
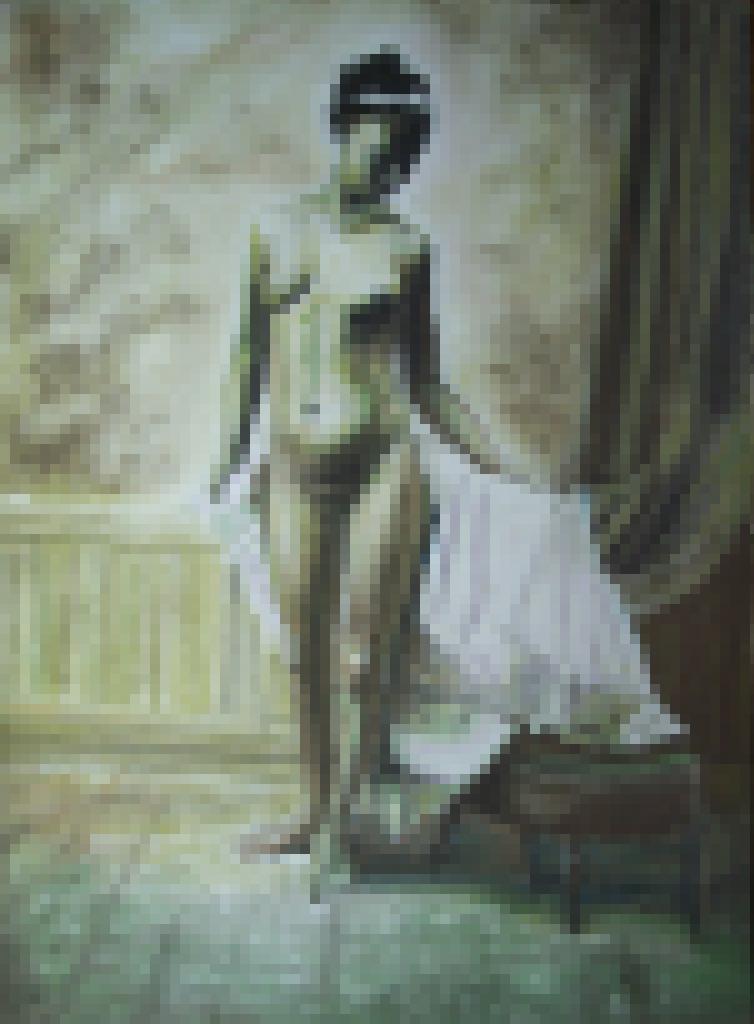
Algorithm 9: Median filter
This filter is based on c.m. filter also but instead of calculate central point in filter window (in aperture) with special formula, it's sort all points and use central point of sorted points and central point of window. So, if there is big impulse in window's central point then it will be replaced by "median" point. It's good to filter "salt and piper" noise.see:
test09.scm
(load "imsh.scm") (define b (img_open "in/ngirl.jpg")) (img_med b 9) (img_save b "out/med.jpg")
Input images:
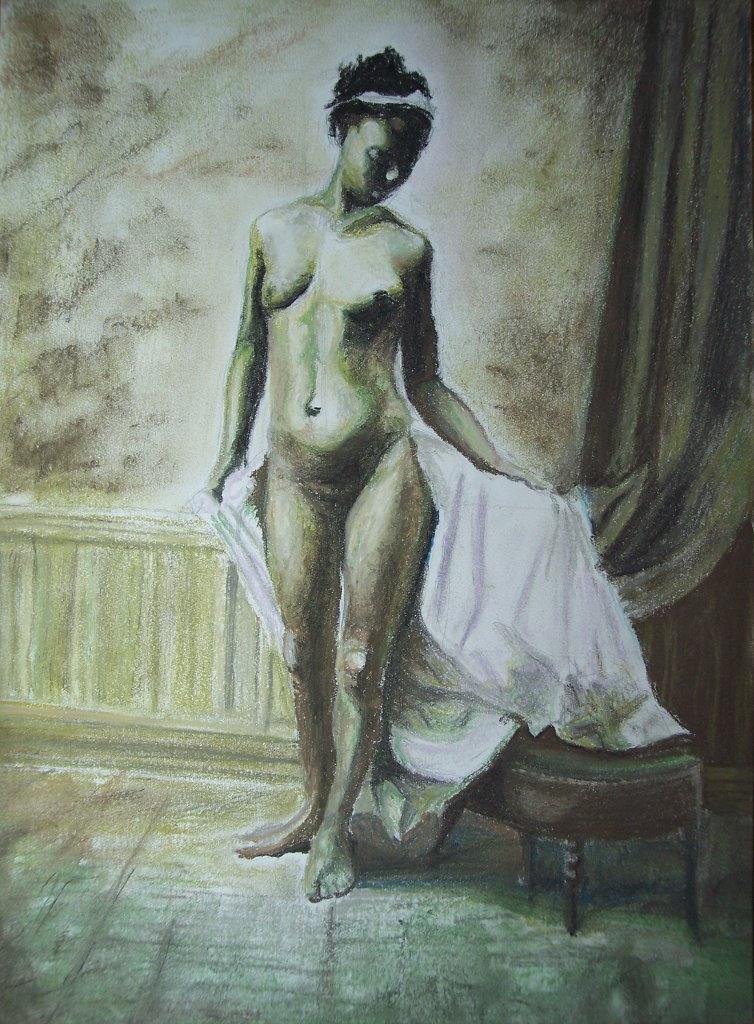
Output images:
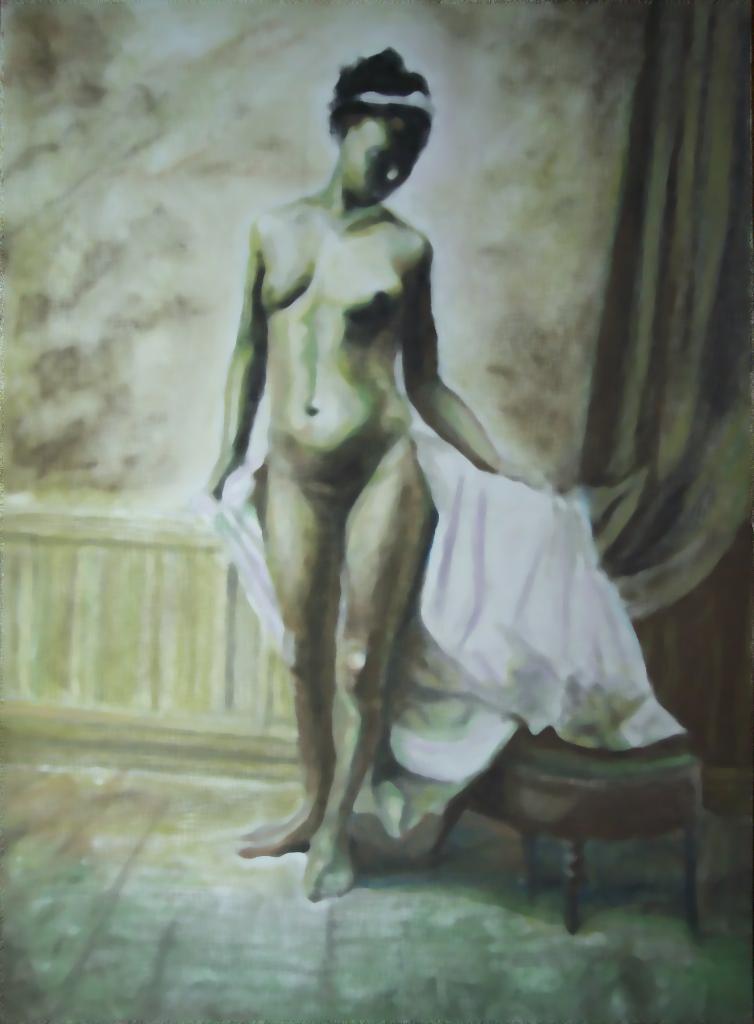
Algorithm 10: Dilation + erosion
This test makes dilation and erosion. Dilation is - application of structural element (like kernel in c.m.) on binary image to get more "fat" image. See more here. Erosion is - application of structural element (like kernel in c.m.) on binary image to get more "thick" image. See more here.see:
test10.scm
(define b1 (img_open "in/foo.jpg")) (img_bw b1 50) (define matrix1 '(1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1)) (define matrix2 '(1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1)) (img_bindilate b1 matrix1) (img_save b1 "out/bindilate.jpg") (define b2 (img_open "in/boo.jpg")) (img_bw b1 50) (img_binerode b2 matrix2) (img_save b2 "out/binerode.jpg")
Input images:
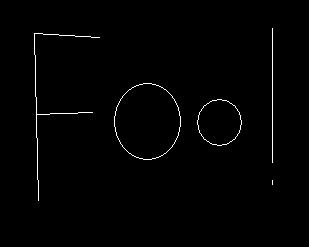
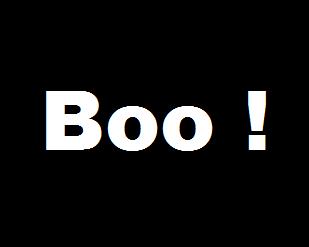
Output images:
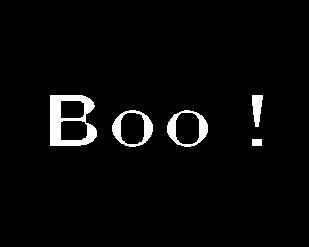
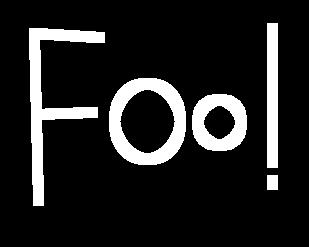
Algorithm 11: More complex dilation + erosion
This test runs dilation and erosion too, but on more complex input image.see:
test11.scm
(define b (img_open "in/thing.jpg")) (img_bw b 50) (define matrix '(1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1)) (img_binerode b matrix) (img_bindilate b matrix) (img_save b "out/open.jpg") (define b (img_open "in/thing.jpg")) (img_bw b 50) (define matrix '(1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1)) (img_bindilate b matrix) (img_binerode b matrix) (img_save b "out/close.jpg")
Input images:
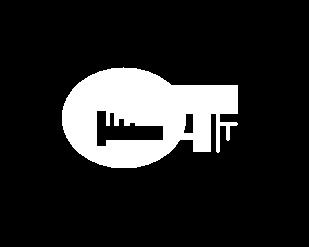
Output images:
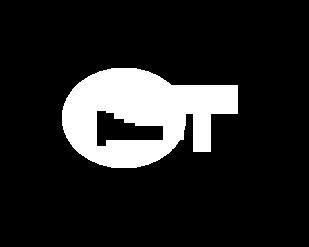
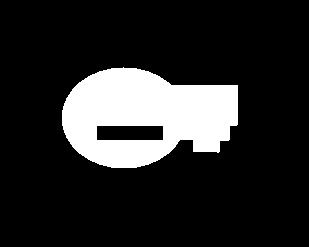
Algorithm 12: Edge detection on bold font
This test runs edge-detection algorithm with c.m. on bold weight font. Result looks interesting and with coloring can get base for neon effect (need also shadowing)see:
test12.scm
(load "imsh.scm") (define b (img_open "in/boo.jpg")) (img_conv b edge-matrix 3 60) (img_bw b 50) (img_save b "out/booedge.jpg")
Input images:
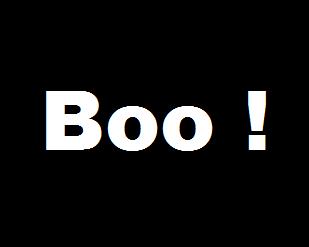
Output images:
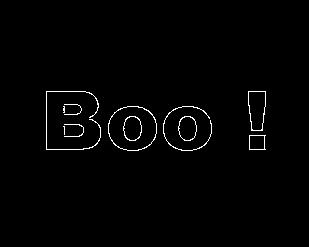
Algorithm 13: Set operations
This test shows set operations but on image: OR, XOR, AND, NOT, SUB (complement).see:
test13.scm
(define b1 (img_open "in/a.jpg")) (define b2 (img_open "in/b.jpg")) (img_bw b1 50) (img_bw b2 50) (img_binand b1 b2) (img_save b1 "out/and.jpg") (define b1 (img_open "in/a.jpg")) (define b2 (img_open "in/b.jpg")) (img_bw b1 50) (img_bw b2 50) (img_binor b1 b2) (img_save b1 "out/or.jpg") (define b1 (img_open "in/a.jpg")) (define b2 (img_open "in/b.jpg")) (img_bw b1 50) (img_bw b2 50) (img_binxor b1 b2) (img_save b1 "out/xor.jpg") (define b1 (img_open "in/a.jpg")) (define b2 (img_open "in/b.jpg")) (img_bw b1 50) (img_bw b2 50) (img_binsub b1 b2) (img_save b1 "out/sub.jpg") (define b (img_open "in/a.jpg")) (img_bw b 50) (img_binnot b) (img_save b "out/not.jpg")
Input images:
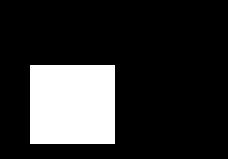
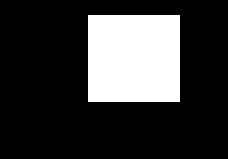
Output images:
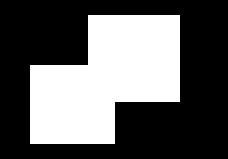
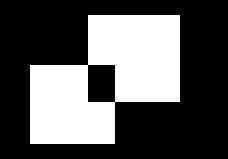
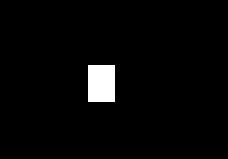
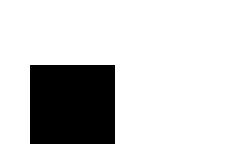
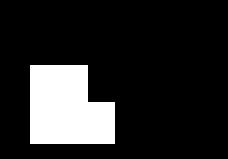
Algorithm 14: Morphological edge detection
Edges can be detected also with morphological operations. Edge := IMG0 `sub` ERODE(IMG0). SUB - is substraction/complements.see:
test14.scm
(define b1 (img_open "in/boo.jpg")) (define b2 (img_open "in/boo.jpg")) (img_bw b1 50) (img_bw b2 50) (define matrix '(0 1 0 1 1 1 0 1 0)) (img_binerode b2 matrix) (img_binsub b1 b2) (img_save b1 "out/morphedge.jpg")
Input images:
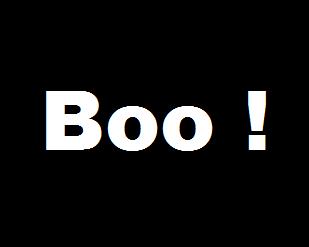
Output images:
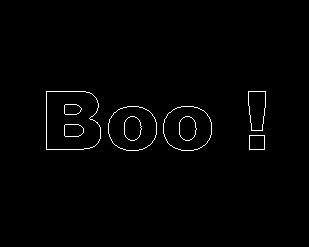
Algorithm 15: Dithering
Dithering is the algorithm for preparing of image to black-white printing (old newspapers, old books) or for old displays systems. Here is 2 different implementations: * general purpose with using of matrixes defined in imsh.scm library * special dithering algorithms.see:
test15.scm
(load "imsh.scm") (define i (img_open "in/stalin.jpg")) (img_gendith i sierra-dith-matrix) (img_save i "out/dithgen_sierra.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i sierra2-dith-matrix) (img_save i "out/dithgen_sierra2.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i sierralow-dith-matrix) (img_save i "out/dithgen_sierralow.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i floyd-dith-matrix) (img_save i "out/dithgen_floyd.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i falsefloyd-dith-matrix) (img_save i "out/dithgen_falsefloyd.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i jarvis-dith-matrix) (img_save i "out/dithgen_jarvis.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i stucki-dith-matrix) (img_save i "out/dithgen_stucki.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i atkinson-dith-matrix) (img_save i "out/dithgen_atkinson.jpg") (define i (img_open "in/stalin.jpg")) (img_gendith i burkes-dith-matrix) (img_save i "out/dithgen_burkes.jpg") (define i (img_open "in/stalin.jpg")) (img_dith i 0) (img_save i "out/dith_atkinson.jpg") (define i (img_open "in/stalin.jpg")) (img_dith i 1) (img_save i "out/dith_floyd.jpg") (define i (img_open "in/stalin.jpg")) (img_dith i 2) (img_save i "out/dith_ordered.jpg") (define i (img_open "in/stalin.jpg")) (img_dith i 3) (img_save i "out/dith_random.jpg")
Input images:
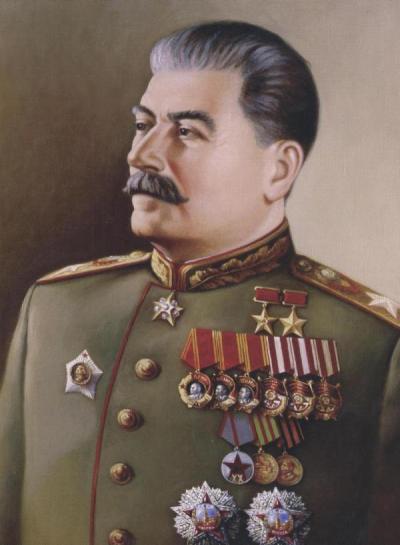
Output images:
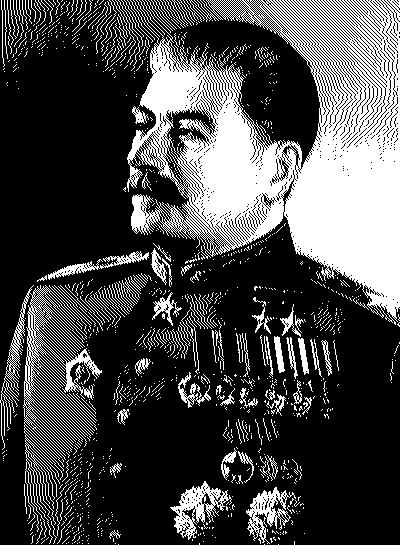
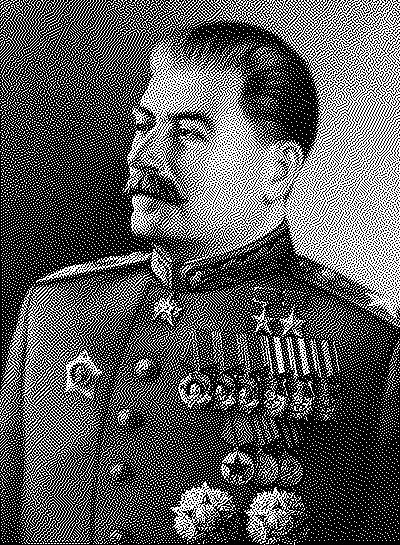
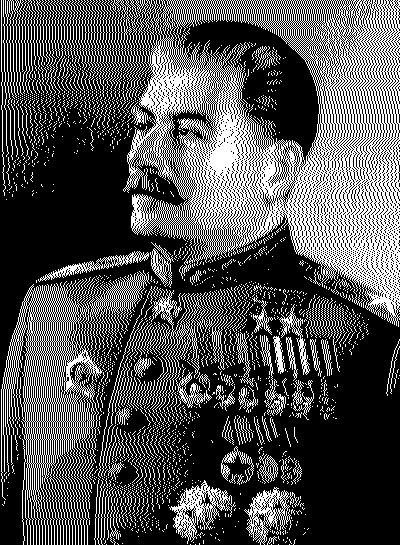
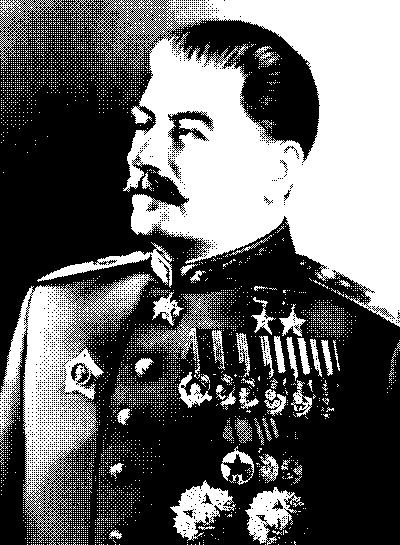
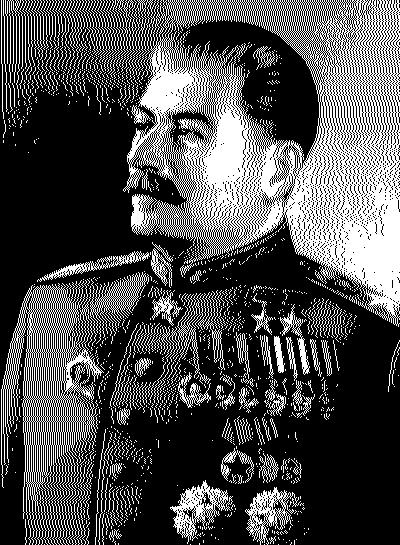
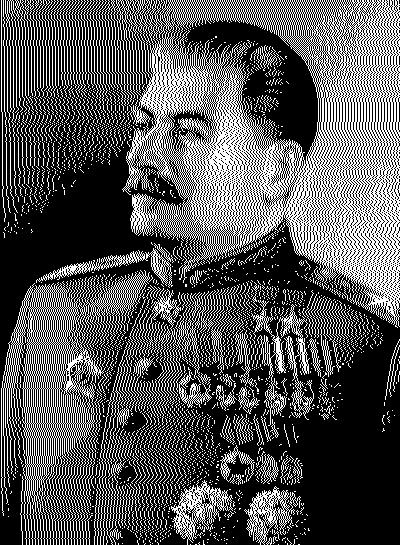
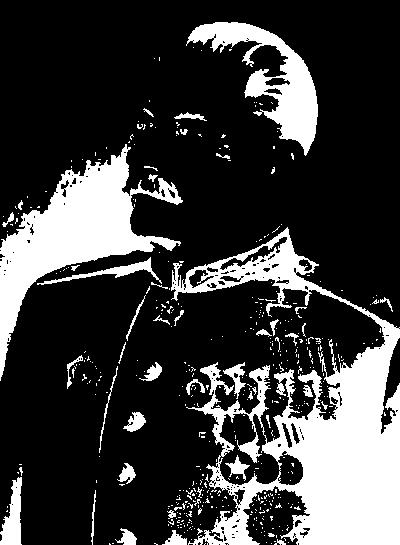
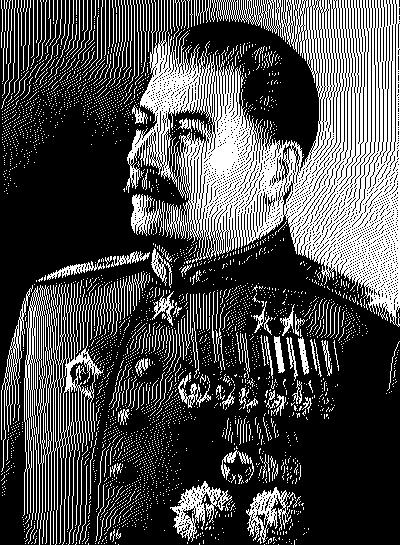
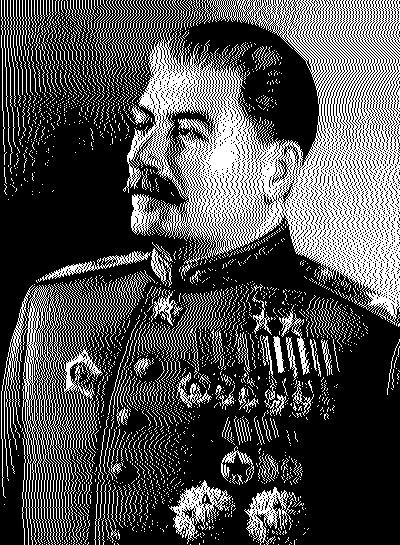
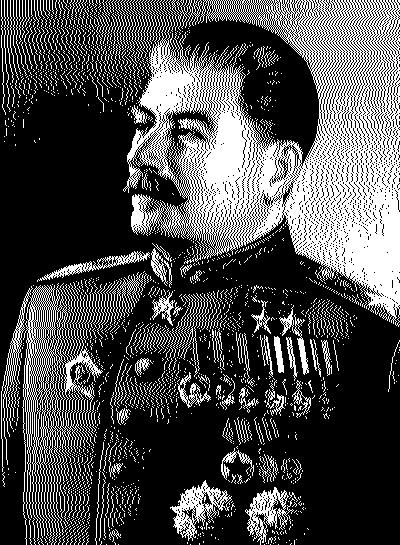
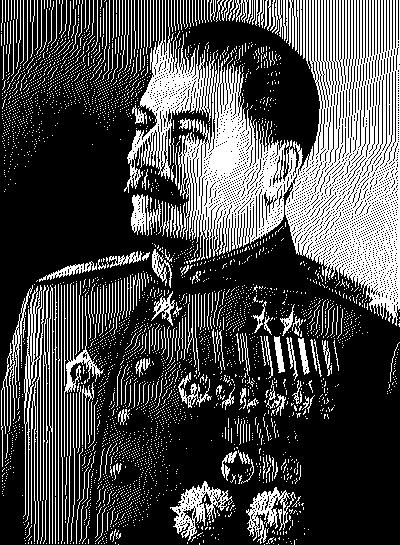
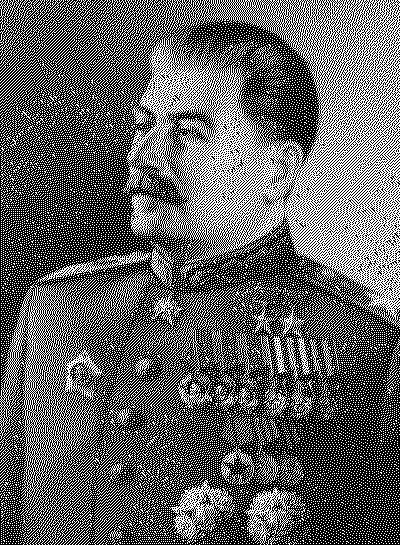
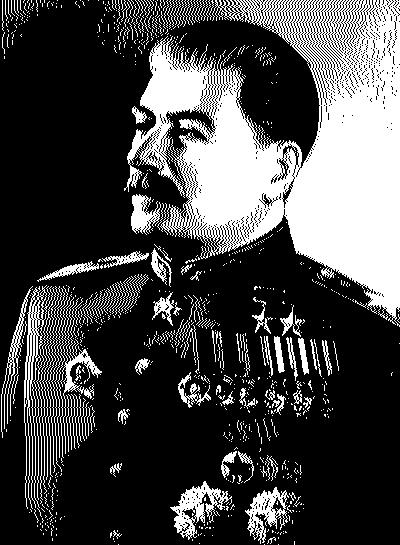
Комментариев нет:
Отправить комментарий
Thanks for your posting!